Code Snippets
/*-- Unit Listing Dual Images --*/
.unit-list .img2 { display: none; }
.unit-list .favorite-container { left: 12px; right: auto; }
@media (min-width: 1600px) {
.unit-list .listMode .unit-media {flex: 0 0 575px;}
.unit-list .listMode .unit-media img {width: 270px;border-radius: 4px;}
.unit-list .listMode .img2 { display: inline-block; margin-left: 15px; }
.unit-list .listMode .unit-pricing {flex: 0 0 280px;padding: 0 0 0 30px;}
}
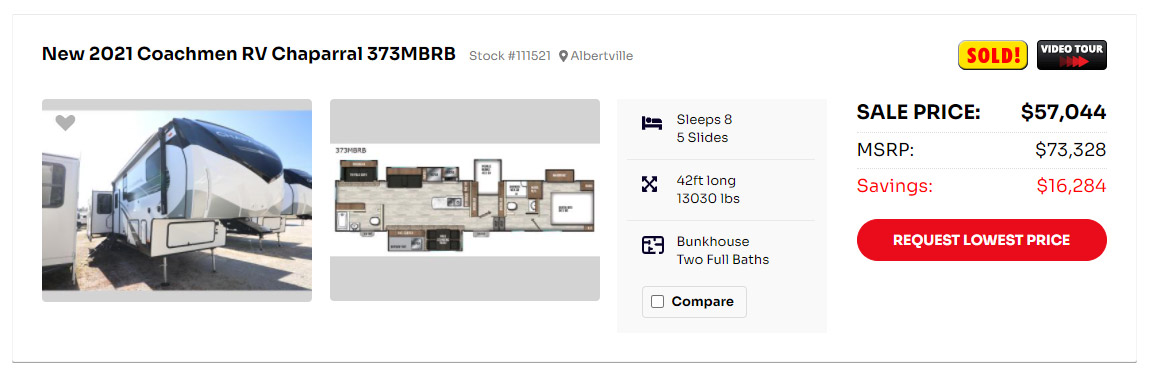
// MOVE SEARCHBAR
<script>
function move_searchbar() {
if ($(window).width() <= 991) {
$('#top-search-container').insertAfter($('#main-nav'));
} else {
$('#top-search-container').insertAfter($('#rv-types'));
}
};
move_searchbar();
</script>
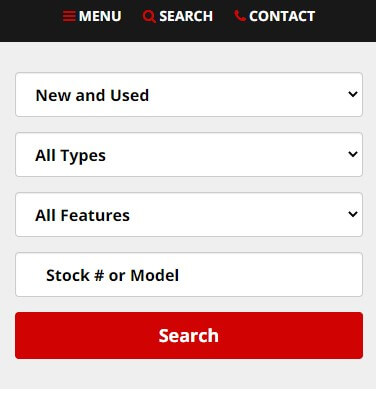
<div class="marquee"><marquee><span>RV Parts....</span> <span>RV Accessories....</span><span>We carry propane....</span><span>We will ship globally....</span></marquee>
</div>
<script>
var row = $('marquee span');
for(var i = 0; i < 10; i++ ) {
$('marquee').append(row.clone())
}
</script>

.home-featured #slideshowWrap .photoContainer:after,
.unit-list .featured-unit .unit-media-wrapper:after,
.unit-detail-v2.featured-unit .detailMediaPhotoPlayer ul.slides > li:after { content: url(https://assets-cdn.interactcp.com/beckleysrvs/images/corner-tab-featured-unit.png); position: absolute; top: 0; left: 0; z-index: 999; }
.unit-list .unit-media-wrapper { position: relative; overflow: hidden; }
.unit-detail-v2.featured-unit .detailMediaPhotoPlayer ul.slides > li { overflow: hidden; }
.unit-list .unit-media-wrapper:after { left: -42px; top: 18px; }
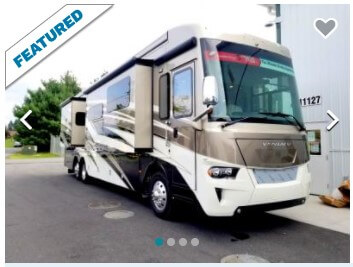
.home-featured #slideshowWrap .photoContainer:after,
.unit-list .featured-unit .unit-media-wrapper:after,
.unit-detail-v2.featured-unit .detailMediaPhotoPlayer ul.slides > li:after { content: 'HOT DEAL'; position: absolute; display: block!important; top: 22px; left: -46px; background: #d7062b; font-family: 'Montserrat', sans-serif; color: #fff; font-weight: 700; font-style: italic; padding: 6px 45px 5px 56px; transform: rotate(-35deg); z-index: 999; }
.unit-list .unit-media-wrapper { position: relative; overflow: hidden; }
.unit-detail-v2.featured-unit .detailMediaPhotoPlayer ul.slides > li { overflow: hidden; }
.unit-list .unit-media-wrapper:after { left: -45px; top: 20px; }
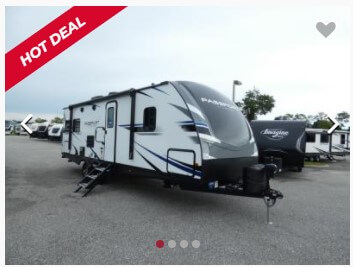
ul.list-checks { margin: 0 0 30px 0; list-style: none; padding: 0; }
ul.list-checks li { position: relative; padding-left: 25px; }
ul.list-checks li:after { content: '\F00C'; font-family: 'fontAwesome'; position: absolute; top: 0; left: 0; color: #242817; }
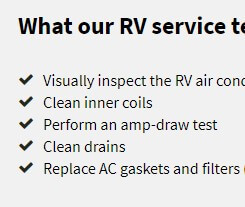
<script>
$(function(){
$('#home-featured-rvs .unit').each(function(){
$(this).find('.pricingContainer').append('<span class="btn btn-primary">SEE DETAILS</span>');
});
});
</script>
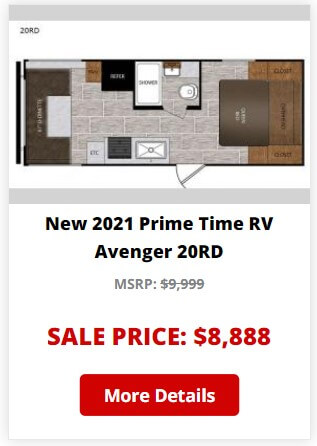
"Call Today!" Link
Adds a link and phone number (based on lot) to each unit on listing and detail pages. Edit link text and styling to match request. Add task nubmer in comment if applicable. Reference: Great Escapes RV
Add script to main node > Developer tab > Page Footer Scripts:
<script>
$( document ).ready(function() {
// Add phone link to units on listing pages
$('.unitList .unit .detailsContainer').each(function () {
var phone = $(this).data('phone');
$(this).find('.unit-price-wrapper').after('<a href="tel:'+ phone +'" class="phone-link"><span>Call Today!</span> '+ phone +'</a>');
});
// Lazy loading
$('.ajax-unit-list').on('unit-loaded', function(e, unit) {
var phone = $(unit).find('.detailsContainer').data('phone');
$(unit).find('.unit-price-wrapper').after('<a href="tel:'+ phone +'" class="phone-link"><span>Call Today!</span> '+ phone +'</a>');
});
});
</script>
Add script to dynamic-inventory-detail > Developer tab > Page Footer Scripts:
<script>
$( document ).ready(function() {
// Add phone link to detail pages
var phone = $('.DetailPanel').data('phone');
$('.DetailPanel .unit-price-wrapper').append('<a href="tel:'+ phone +'" class="phone-link"><span>Call Today!</span> '+ phone +'</a>');
});
</script>
(Optional) Add CSS to main node > Developer tab > Site Stylesheet (add underneath "Site-Specific Styling"):
/* Phone link */
.unit-pricing .phone-link, .unit-price-wrapper .phone-link { display: block; margin: 20px 0 0; text-align: center; color: #000; font-size: 15px; }
.unit-pricing .phone-link span, .unit-price-wrapper .phone-link span { font-weight: 800; }
.unit-pricing .phone-link:hover, .unit-price-wrapper .phone-link:hover { text-decoration: underline; cursor: pointer; }
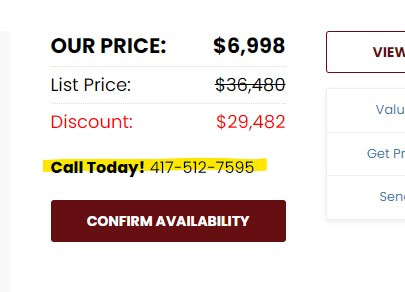
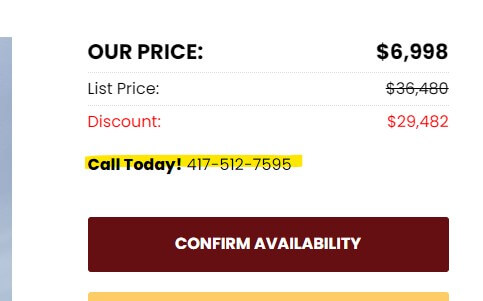
Percent Off
Calculates percentage off if unit has a msrp and sale price, then adds it under the msrp on listing (including lazy load) and detail pages.
SRP - main node > Developer tab > Page Footer Scripts:
<script>
// % OFF on units with MSRP and Sale Price
var addPercentOff = function(u) {
var msrp = $(u).find('.detailsContainer').data('msrp');
var sale = $(u).find('.detailsContainer').data('saleprice');
if (typeof msrp !== 'undefined' && typeof sale !== 'undefined') {
msrp = parseInt(msrp.replace('$','').replace(/\,/g,''));
sale = parseInt(sale.replace('$','').replace(/\,/g,''));
if (msrp > 0 && sale > 0) {
var percent = Math.round((1 - (sale / msrp)) * 100);
$(u).find('.regPrice').after('<li class="percentOff">'+percent+'% OFF</li>');
}
}
};
// Listing pages
$('.unit-list .unit').each(function() {
addPercentOff($(this));
});
// Listing pages lazy loading
$('.ajax-unit-list').on('unit-loaded', function(event, item) {
addPercentOff(item);
});
</script>
VDP - dynamic-inventory-detail > Developer tab > Page Footer Scripts:
<script>
// % OFF on units with MSRP and Sale Price
$(function() {
var msrp = $('.DetailPanel').data('msrp');
var sale = $('.DetailPanel').data('saleprice');
if (typeof msrp !== 'undefined' && typeof sale !== 'undefined') {
msrp = parseInt(msrp.replace('$','').replace(/\,/g,''));
sale = parseInt(sale.replace('$','').replace(/\,/g,''));
if (msrp > 0 && sale > 0) {
var percent = Math.round((1 - (sale / msrp)) * 100);
$('.DetailPanel .PriceText').after('<span class="percentOff">'+percent+'% OFF</span>');
}
}
});
</script>
(Optional) Add CSS to main node > Developer tab > Site Stylesheet (add underneath "Site-Specific Styling"). Edit as needed:
.percentOff { display: block; color: #028c3c; font-weight: 700; font-size: 17px; }